20 Jul 2020
For details about securing Firefox, see the equivalent Firefox post
Firefox is my preferred browser, but Chrome is currently the clear marketshare leader among browsers. As of early 2020, around 65% of browser usage is Chrome. In addition, at least a couple of other popular web browsers, the Brave browser and the new Microsoft Edge browser, borrow from Chrome’s codebase.
Chrome vs. Chromium
First, we must clarify the difference between the Chrome browser and the Chromium browser, because a lot of confusion exists between the Google’s two. open source project that is the foundation for Google Chrome. Chrome uses the Chromium source code as well as Google’s proprietary modifications. Therefore, while Chromium is licensed as open source software, Google Chrome is licensed as proprietary freeware.
Can’t have Chrome without the Google
If you are concerned about privacy as well as security, remember that Google creates the Chrome browser. Google makes the majority (~80%) of its revenue from advertisements. Because targeted advertising is more valuable, Google has incentive to gather data on web users to better target advertisements. By default, Chrome has some built-in features that can reduce a user’s privacy. Fortunately, there are several Chromium project forks that are built for improved privacy. For example, “Ungoogled Chromium” removes google dependencies in the Chromium code, in addition to other privacy improvements. If you choose to use this Chromium fork, I highly recommend reviewing the FAQ, as it explains how to overcome common hurdles, such as installing extensions from the Chrome web store. While the rest of this article will reference Chrome, Chromium can benefit from the same security improvements.
Don’t login to Chrome
This first suggestion is obvious if you wish to minimize Google’s data collection. Logging into the Chrome browser for extended periods can provide Google with insight into your web browsing habits. Note that in the most recent Chrome releases, you are automatically logged into Chrome when you login to Google services, such as Gmail, so logging in to Chrome can happen without you even noticing.
Add features to Chrome shortcut
Some of the security features in Chrome cannot be modified in a menu of settings, but instead need to be arguments provided to Chrome when Chrome is started. To provide arguments to Chrome, you need to find the shortcut that you use to start Chrome, right click this shortcut, and select “Properties” (or “Edit”, depending on your OS), and change the path or command that starts Chrome. Several examples of features that can be added with this approach are:
- Opening Chrome in private browsing mode (AKA “incognito” in Chrome), where no history is saved, requires passing an argument to chrome, specifically
-incognito
- To use DNS over HTTPS, use the argument
--enable-features="dns-over-https<DoHTrial" --force-fieldtrials="DoHTrial/Group1" –force-fieldtrial-params="DoHTrial.Group1:server/https%3A%2F%2Fcloudflare-dns%2Ecom%2Fdns-query/method/POST"
- If you wish to disable canvas fingerprinting entirely (which might not be the best approach, compared to spoofing a false canvas fingerprint value), you can use the
--disable-reading-from-canvas
argument
After deciding which features you want to enable, you may end up with a shortcut to start Chrome that contains a list of command arguments such as the following:
/usr/bin/google-chrome-stable %U -incognito --enable-features="dns-over-https<DoHTrial" --force-fieldtrials="DoHTrial/Group1" --force-fieldtrial-params="DoHTrial.Group1:server/https%3A%2F%2Fcloudflare-dns%2Ecom%2Fdns-query/method/POST" --disable-reading-from-canvas
Extensions
Fortunately, many of the browser extensions that I am familiar with in Firefox are also found in Chrome. Below is a list of Chrome extensions I prefer, but I have chosen most of these because I am familiar with using them on Firefox.
Use chrome://flags??
The most confusing part of my exploration of improving Chrome’s security is the lack of information about privacy-related and security-related flags that can be set on the special chrome://flags
page. I assumed that this is the Chrome equivalent of Firefox’s about:config
, but it appears to allow for much less granular control. I couldn’t even come up with a list of 5 good security features to enable on this page, so if I’m missing something obvious, please reach out and let me know! My current conclusion is that Google is targeting the average user who wants technology that “just works”, even at the cost of privacy, and therefore they don’t see the value in providing detailed configuration settings.
Other best practices
This list only is only a summary of the many ways that your browser can be secured. For good measure, I will list a few miscellaneous suggestions that can also help for various reasons:
- Disable Chrome Privacy Sandbox settings as described here
- Use strong, unique passwords and change them at least once per year. Weak or compromised passwords are still one of the most common ways that security is broken. If your password is leaked (for example, if all the accounts for a certain website were leaked onto the internet), this limits the timeframe when another person may be able to access your account.
- If you use Chrome on your mobile device, secure your mobile browser with the same steps used here. Some of these settings require a different process to set on your mobile device, such as always opening Chrome in private browsing (AKA incognito) mode.
- Change your default search engine to DuckDuckGo. Since Google attempts to target ads by gathering data on users, the privacy-centric DuckDuckGo is a great choice for anyone wishing for a little less Google in their life. Or if you want an alternative to DuckDuckGo, you could try Searx.
- Test your browser to make sure your expectations are aligned with reality. Some useful website are Panopticlick (to check your browser’s fingerprint), Cloudflare’s ESNI checker (to check DNS over HTTPS), and BrowserLeaks (to check a variety of browser settings).
Overall, I’d still suggest Firefox as the best web browser for someone who wants to customize their browser for security and privacy needs. The lack of easy configuration for multiple useful settings (always private browsing, DNS over HTTPS) create a privacy and security drawback in Chrome. After all, there is a reason why the Tor browser is built on Firefox. If you’re interested in switching to Firefox, check out the first article explaining how to secure Firefox and compare those features with Chrome’s.
06 Jul 2020
The Advanced Web Security series looks beyond the OWASP Top 10 web application security risks. For the full list of articles in this series, visit this page.
When you are browsing the web, most of the data sent to your browser uses the HTTP protocol. WebSockets are a newer feature from HTML5 that does not use HTTP, and therefore they are often overlooked. Luckily, WebSockets are simpler than HTTP, so they are easier to check for common vulnerabilities.
Observing WebSocket Traffic
If you are using a web proxy tool like Burp Suite or OWASP ZAP, WebSocket traffic is shown in a separate tab from where HTTP traffic is shown. Below are screenshots showing where to find this tab. Note that in ZAP, the tab might only appear when WebSocket traffic exists.
Figure 1: The OWASP ZAP WebSockets tab
Figure 2: The Burp Suite WebSockets tab
The website http://websocket.org/echo.html can also be used to run a simple WebSocket connection test.
Vulnerability #1: Unencrypted WebSockets
WebSockets do not use HTTP, but instead use their own protocol. WebSocket communications are sent to an address that starts with ws:// or wss://, similar to how the HTTP protocol uses http:// and https://. Communications to an address starting with ws:// are not encrypted, which is a security concern. Instead, communications should be sent to an address starting with wss://, which encrypts the traffic using TLS, just like HTTPS.
Vulnerability #2: Sensitive data leak
WebSockets can reveal sensitive data that the server should not be sending to users. For example, WebSocket traffic may reveal internal server details, hidden features in the web application, or data about other user accounts (such as usernames). Leaked information may be a security concern in itself, but the information may also assist another attack elsewhere in the web application.
Vulnerability #3: Insecure authentication
The WebSocket protocol does not implement authentication. If the previous sentence does not sound a warning alarm in your mind, it should! The lack of built-in protocol-level authentication means the web application developer must make decisions about how to authenticate WebSocket connection requests, and custom authentication solutions often have vulnerabilities. The authentication process for creating new WebSocket connections should always be tested for vulnerabilities. The server may allow WebSocket connections without valid tokens, with a username that does not match the current token, or other authentication implementation errors.
Vulnerability #4: Cross Site WebSocket Hijacking (CSWSH)
The same-origin policy is a security feature that websites use to prevent Cross-Site Request Forgery (CSRF). However, WebSockets are not protected by the same-origin policy. The server must protect WebSocket connection requests against a CSRF type of attack by using 1. a CSRF token for each WebSocket connection request and 2. the “Origin” header in the WebSocket connection request. If these solutions are not implemented, a Cross Site WebSocket Hijacking (CSWSH) vulnerability may exist. This can be a serious vulnerability, as this $12,500 bug bounty shows.
Vulnerability #5: Conventional risks
WebSockets can provide another chance to test other conventional attacks. XSS, SQLi, IDOR, and other vulnerabilities may be exploited using WebSockets. Exploiting these vulnerabilities using WebSockets requires knowledge of these other risks, and therefore may take time and understanding to fully test. Understanding the data that is being transmitted over WebSockets is a key to exploiting these vulnerabilities.
Vulnerability #6: Reverse proxy bypass
This may be the newest WebSocket vulnerability in this list. Mikhail Egorov outlined this vulnerability at Hacktivity 2019, and the end result is that an attacker can communicate with private server resources that should not normally be accessible to users. This can be done by modifying HTTP request headers in a WebSocket connection request to unexpected values, such by setting the Sec-WebSocket-Version header to a four digit number. It is also possible to add the “Upgrade: websocket” request normal HTTP requests to check if the server treats the request as a WebSocket connection request. Based on Mikhail’s initial findings, only the Envoy proxy (version 1.8.0 and before) and the Varnish proxy were found to be vulnerable. I expect more research like this will be done soon and reveal further findings.
References
CSWSH introduction: https://www.christian-schneider.net/CrossSiteWebSocketHijacking.html
Portswigger Web Academy WebSocket labs: https://portswigger.net/web-security/websockets
Site that allows simple WebSocket connection test: http://websocket.org/echo.html
Mikhail Egorov’s conference talk on WebSocket security: https://www.youtube.com/watch?v=gANzRo7UHt8
22 Jun 2020
This post builds upon the previous Firefox post in this series, which discusses intermediate Firefox security improvements.
Proceed with caution
Why do I consider the Firefox security suggestions below to be “advanced”? First and foremost, some of the settings can and will break your normal web browsing experience. Being able to pinpoint the cause of such trouble, when you encounter it, requires patience and understanding of the underlying parts involved. The second and lesser reason is that I expect only a small minority of Firefox users delve into these more effort-intensive security modifications, which means these are roads less traveled. With that said, let’s look at how to add further layers of security and privacy to Firefox.
about:config changes
You can set thousands of internal configuration settings with the about:config URL in Firefox. This is where many “advanced” Firefox settings can be altered. The best guide I know of summarizing which about:config settings to modify is at privacytools.io, and I suggest using at least the first half of their suggested settings, if not all of them.
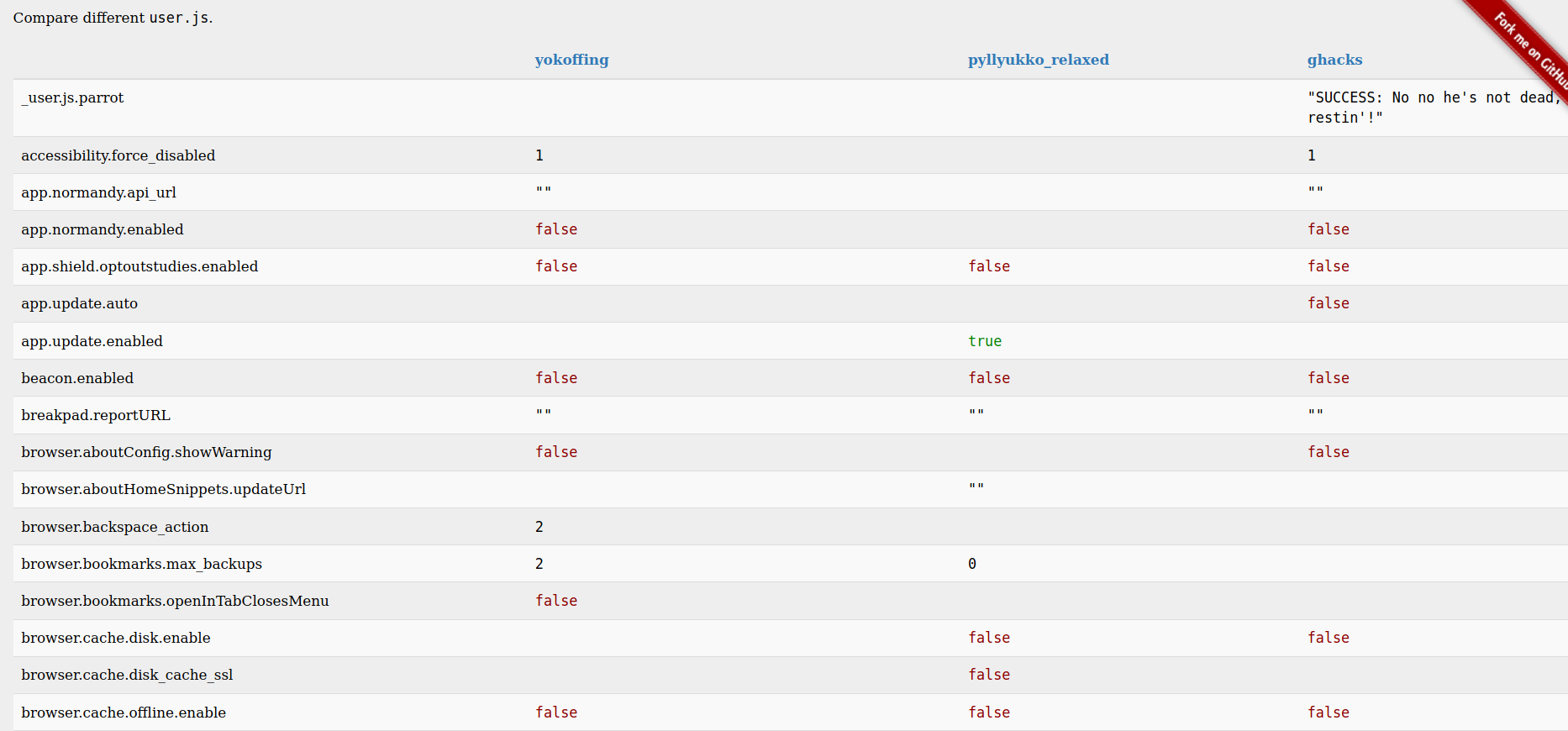
Use a secure Firefox user.js configuration
If you want to modify dozens of about:config settings to values that have already been reviewed by a security-minded community, try using a custom user.js configuration file. The user.js file is read when Firefox starts up and sets various values found on the about:config page. The number of configuration values set in these custom user.js files can be a bit daunting to examine, so I would recommend either testing these in newly created profiles or skimming the comparison of popular user.js projects. The links to the corresponding user.js projects are also found on that page. Anything that the user.js file can configure can also be configured manually using about:config. So if using an intense user.js file will impact your web browsing activities too much, consider either a simplified user.js file like SecureFox or manually configuration of important settings. The settings below are ones which I have only seen set in user.js files rather than tutorials explaining manual modification of about:config:
- Disabling insecure TLS cipher suites
- Disabling WebAssembly and asm.js
- Disabling DOM features
Securing your mobile browser
If you have a smartphone, odds are you have a web browser installed on it. But have you applied the same security hardening on your phone that you use on your primary computer? Some of the features in the mobile version of Firefox are set differently than the desktop version (such as, at the time of writing, DNS over HTTPS).
Test your configuration
Regardless of how secure you want your web browser, it is important to confirm that the security improvements you think you have applied are actually working. Some tests can be run locally, such as by using a web proxy (such as Burp Suite or OWASP ZAP) or monitoring your network traffic. However, an easier approach is to use websites that test a certain aspect of your browser’s security - a few are below:
Leverage extensions
You are no doubt aware that Firefox has an extensive collection of extensions that can be installed, but below are a few that may add extra protection:
Bonus points
Some of the extensions mentioned, including uBlock Origin, have enough settings to necessitate a wiki! Bonus points if you use uBlock in “Hard Mode” or fully utilize the full featureset of some of the other tools mentioned (such as NoScript). Please check out additional information sources if you’re serious about securing your browser – Firefox has an extremely large number of features. And if you’re still feeling insecure after all these measures, it might be time for you to investigate the Tor project…
08 Jun 2020
Web browsers are the most common window to the internet, but default browser settings are not designed for security or privacy. This post is the first in a series exploring ways to improve your web browser’s security configuration.
To start, what security and privacy risks can a web browser play a part in? Unfortunately, there are many, including:
- Phishing attacks: resulting in stolen credentials
- Drive-by downloads: resulting in ransomware or malware that can ruin your computer
- Man-in-the-middle attacks: resulting in a third-party observing your communications, compromising accounts and sensitive data
- Cryptojacking: resulting in your computer slowing down, and increasing your electricity costs while others profit
- Third-party trackers: resulting in creepy ads, your personal data being warehoused by many companies, increasing the risk of identity theft
These risks can be reduced by you! You accept these risks if you do not secure your browser properly. There are other risks that you can’t do much about, like a government agency getting hacked or a company unknowingly giving your data to the third parties, but it makes sense to take control of the risks you can manage. So, how can you secure your browser?
Preventing phishing attacks
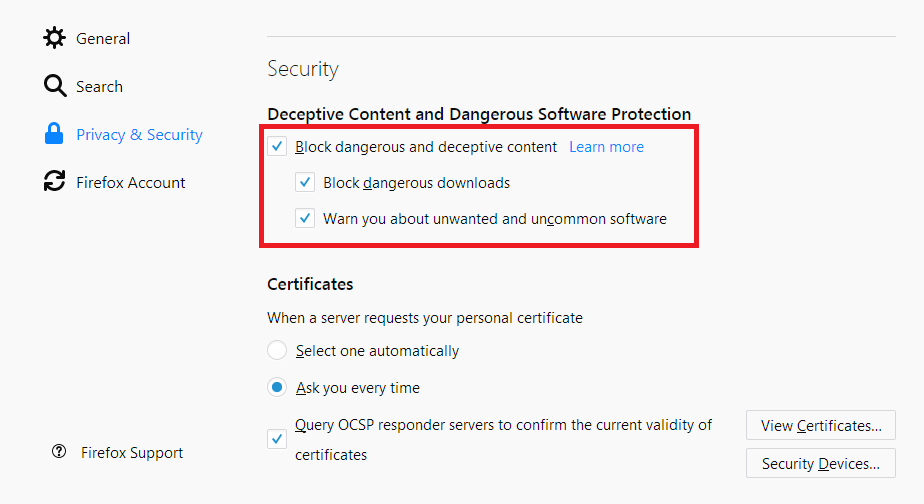
Phishing is an attack where an attacker gets your sensitive credentials, such as your banking password, by tricking you to login to a fake website. Firefox has phishing protection built-in – you just need to activate it. The above screenshot shows where to find the setting in the Firefox’s “Preferences” area, but the full instructions are on Mozilla’s website.
For additional protection, the antivirus maker BitDefender published a Firefox extension that can help prevent phishing by rating website links. I haven’t used this yet, but it could be useful if your are particularly concerned about this type of attack.
Preventing drive-by downloads
Drive-by downloads can force your browser to download malicious files without your knowledge, resulting in your viruses, malware, and other nasty results. Drive-by downloads prey on software bugs in your browser, usually in common pieces of software. The best thing you can do to reduce the number of browser software bugs is to disable software you don’t need. Specifically, Firefox plugins such as Java, Flash Player, Adobe Reader, or Quicktime should be disabled or removed completely. Less code means fewer bugs. Another way to reduce the bugs in your code is by keeping your browser updated. In general, if a piece of software offers you an update, you should install the update!
Man-in-the-middle Attacks
A man-in-the-middle attack exists when an attacker controls a part of the connection between you and a website you visit, and therefore the attacker can see the data that you are sending to the website. For example, if you are connected to a free public Wi-Fi in a hotel, an attacker may unexpectedly control this Wi-Fi network. The best way to protect yourself is to use encrypted connections. The HTTPS Everywhere extension forces websites to use HTTPS, a form of encrypted connection, if the website supports this technology. However, HTTPS normally only protects the data you send to websites, meaning a man-in-the-middle attack could still see the URLs you are visiting. You can add further protection by enabling DNS over HTTPS, which will encrypt the URLs that you are visiting. This is another setting you can change in the “Preferences” area of Firefox. Mozilla has a detailed guide showing how to enable DNS over HTTPS.
Preventing Cryptojacking
Cryptojacking is an attack where an attacker uses your computer to mine cryptocurrency to make the attacker money. An attacker can do this by putting cryptomining code into a website, such as through an advertisement. Fortunately, Firefox has built-in cryptojacking protection, seen below, which should be enabled – see Mozilla’s guide explaining how to do that. Going further, perhaps the best single Firefox extension I can suggest to anyone is uBlock Origin, which is my favorite adblocker. This extension alone can protect against several types of attacks listed here.
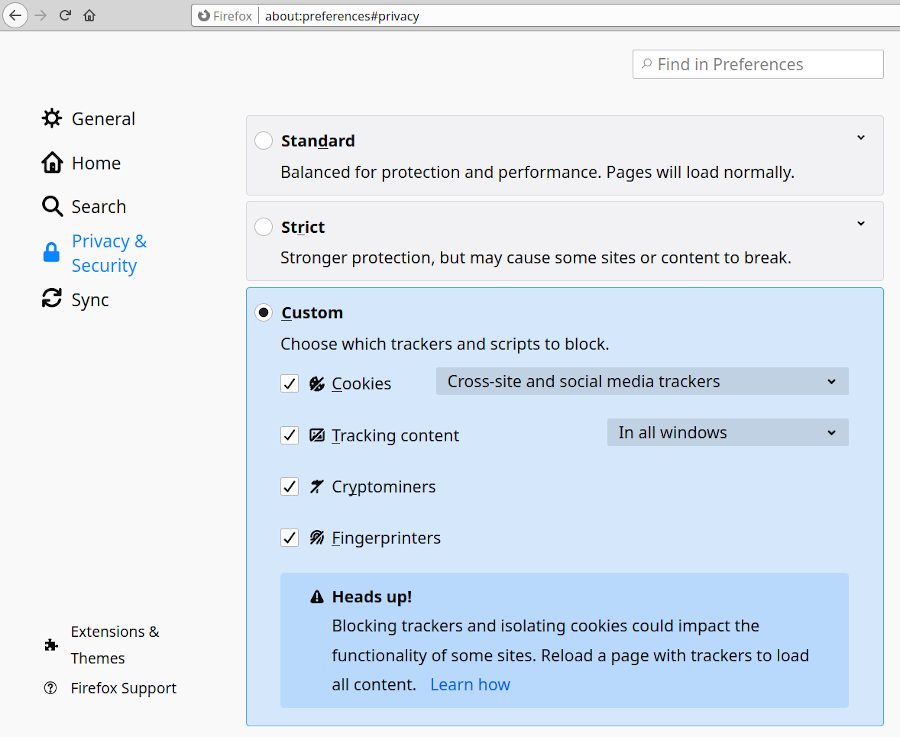
Preventing third-party trackers
Third-party trackers follow you around the internet as you visit different sites, sometimes even following you to other devices (such as your phone), so that they can serve you targeted advertisements. The companies that collect this information from trackers can also sell that data, either to advertisers or malicious users, so it’s best to block these trackers when possible. The first step to blocking trackers is to use Firefox’s built-in protection, seen in the screenshot above. As you may have guessed, Mozilla has a guide on how to do that. To go beyond Firefox’s protections, I suggest using the Cookie Autodelete extension and the Privacy Badger extension to add further layers of protection to block any trackers that slip past the built-in Firefox protections.
Other best practices
This list is only a brief summary of the many ways that your browser can be secured. For good measure, I will list a few other suggestions that can help further:
- Use strong, unique passwords and change them at least once per year. If your password is leaked (for example, if all the accounts for a certain website were leaked onto the internet), this limits the timeframe when another person may be able to access your account.
- Enable Firefox’s breach notifications feature. This will alert you if a website has been publicly hacked and the account information leaked onto the internet.
- Change your default search engine to DuckDuckGo. Knowing that Google makes over 80% of all its income from ads, the privacy-centric DuckDuckGo is a great choice for anyone wishing for a little less Google in their life.
- Using the private browsing feature is always a good idea if you don’t need to save your browsing history. When private browsing is enabled, cookies and other data will automatically be deleted when you close Firefox. This doesn’t make everything you do completely private, but it’s a helpful feature.
If these security tweaks were all common sense for you, be sure to check out the advanced Firefox browser security post for additional security improvements.
25 May 2020
Don’t be afraid if you are no longer considered a kid! I am borrowing the idea from the Feynman Technique, which suggests that the best way to validate understanding of a subject is to try to explain the subject to a middle school student. The idea is that a subject that is not explained in a simple manner is not fully understood. Therefore, if I can’t explain a security topic in a way that can be understood by kids, I don’t understand the topic well. My goal on this blog is to write about security topics in a way that minimizes hand-waving explanations, generalizations, and buzzwords, which are habits that can hide gaps in understanding. Constant learning is crucial in the cybersecurity field because of the amount of new information and research published. This blog will help me to keep pace of these new developments and solidify my knowledge of a wide range of topics. Besides validating my understanding, my hope is that this blog will provide useful information to you in a clear and concise manner.